我们可以在http://tool.chacuo.net/cryptaes 进行在线加密与解密。
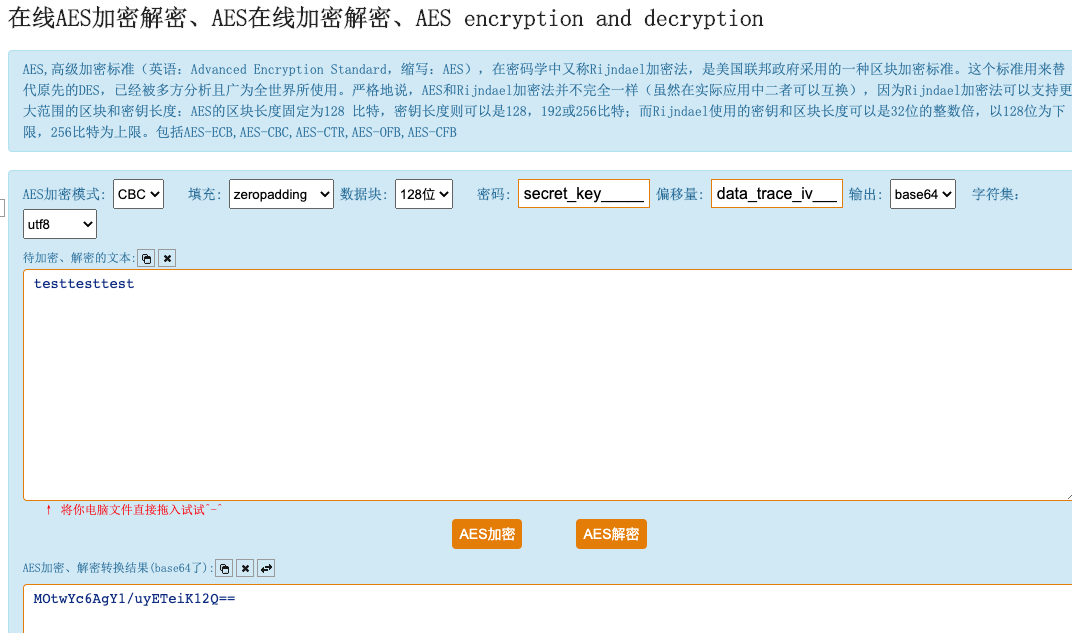
python里面需要安装pycryptodemo(而不是Crypto),之后可以用以下方式进行加密与解密:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52
| from Crypto.Cipher import AES import base64
class PrpCrypt(object): def __init__(self, key, iv): self.key = key.encode('utf-8') self.iv = iv.encode('utf-8') self.mode = AES.MODE_CBC
def encrypt(self, text): text = text.encode('utf-8') cryptor = AES.new(self.key, self.mode, self.iv) length = 16 count = len(text) if count < length: add = (length - count) text = text + ('\0' * add).encode('utf-8') elif count > length: add = (length - (count % length)) text = text + ('\0' * add).encode('utf-8') self.ciphertext = cryptor.encrypt(text) return base64.b64encode(self.ciphertext)
def decrypt(self, text): cryptor = AES.new(self.key, self.mode, self.iv) plain_text = cryptor.decrypt(base64.b64decode(text)) return bytes.decode(plain_text, encoding='utf-8').rstrip('\0')
data_trace_aes_cbc_pc = PrpCrypt('________________', "________________")
def get_encrypt(d): return data_trace_aes_cbc_pc.encrypt(d)
def get_decrypt(e): return data_trace_aes_cbc_pc.decrypt(e)
|